Hey there friend! Let’s dive into API calls – something that sounds super techy but is actually pretty straightforward once you break it down.

Think of it like ordering food from your favorite restaurant except instead of a waiter you’re talking directly to the restaurant’s kitchen (the API) using a coded message.
Ready to level up your dev game? 🚀 This guide breaks down API calls like a pro. Want the cheat sheet to master them? Check out this awesome resource! 💯

Understanding API Calls: It’s Easier Than You Think!
An API call is basically a request you send to a server asking for specific data or to perform a certain action.
The server which hosts the Application Programming Interface (API) processes your request fetches the info (or does the action!) and sends the result back to you.
It’s like a super-efficient messenger service for digital information.
For example when you use a weather app that app is making an API call to a weather data provider to get the current temperature and forecast.
It’s all happening behind the scenes making your life easier.
Think about it like this: you want to see movie times at your local cinema.
Instead of calling them directly you use an app (like Fandango or Atom Tickets). That app makes an API call to the cinema’s system to get the schedule and then neatly displays it for you.
No messy phone calls or confusing websites needed! It’s all about efficiency and automation.
Its really quite amazing isnt it? The power of technology..
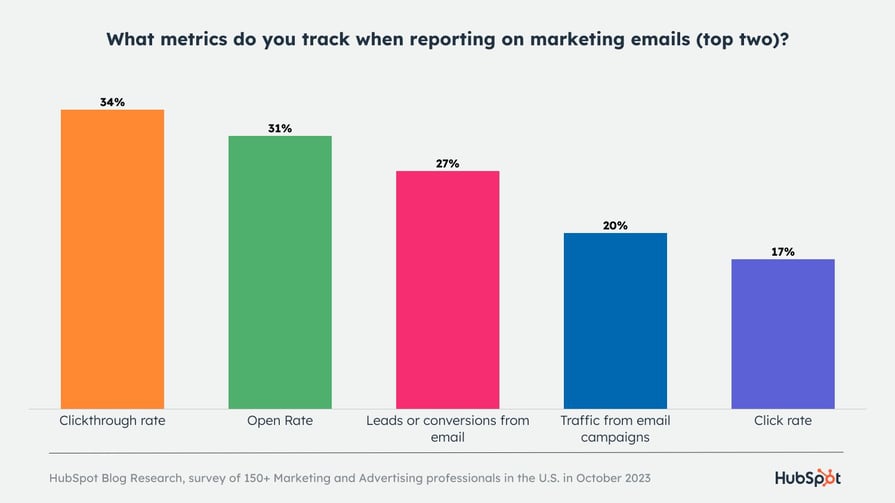
Why Bother with API Calls?
Why go to all this trouble? Because API calls are incredibly powerful tools for developers.
They allow different software systems to communicate and share data seamlessly.
This allows developers to create more dynamic and integrated applications that are more functional for end-users.

It’s all about combining strengths—one system might be great at storing data another at processing images and an API call lets you make them work together beautifully.
And it’s this communication that saves a lot of time and effort!
This kind of streamlined communication lets developers build awesome things that would otherwise be impossible or incredibly time-consuming.
Imagine having to manually input data from one system into another every time you needed to update information.
Nightmare!
Making API Calls: A 5-Step Guide
Now for the practical stuff.
Let’s walk through making an API call in five simple steps.
Don’t worry; it’s less complicated than it sounds.
I promise.
Step 1: Find the API’s Address (URI)
First you need the API’s “address” called a Uniform Resource Identifier (URI). Think of it like a website address but specifically for the API.
This tells your application exactly where to send its request.
Most APIs will provide this information in their documentation – it’s typically a URL (like https://api.example.com
). You can’t send a letter without an address right? Same deal here!
This URI often includes different “endpoints” which are like specific rooms within the API’s building.
Each endpoint handles a particular type of request.

For instance a social media API might have endpoints for getting user profiles posting updates or searching for hashtags.
The documentation will provide a clear map for all the endpoints.
There are many different types of URI’s depending on the type of API your using like REST API’s SOAP API’s GraphQL API’s and many more.

Each of them differ in how they send and receive requests and responses.
Step 2: Choose Your Verb (HTTP Method)
Next you need to choose the right “verb” or HTTP method.

This tells the API what kind of action you want it to perform.
The most common verbs are:
-
GET: This requests data from the server. Think of it as asking “What’s the latest weather?”
-
POST: This sends data to the server typically to create something new (like adding a new user to a database). You might think of this like sending a message to the server asking to add information to its database.
-
PUT: This updates existing data on the server. If you want to update information on the server then this is your method.
-
DELETE: This removes data from the server. If you need to delete something from the server this is what you need.
The verb is crucial; using the wrong one will often result in an error.
The HTTP methods are an essential part of making API calls and depending on the type of request you are making your API call will require a specific HTTP verb.
Choosing the right verb is essential for successful API calls and its important to read your documentation carefully for more information on what type of verb to use.
Step 3: Include Headers (The Important Stuff)
Headers are extra information you send along with your request like adding a note to your letter.
They give the API more context.
Essential headers include:
-
User-Agent
: This identifies your application. It’s like signing your name on the letter. -
Content-Type
: This specifies the format of the data you’re sending (usually JSON or XML). This is like labeling your package to make sure it gets to the right location. -
Accept
: This tells the API what format you want the response in (also usually JSON or XML). This is like the return address on your package so it can be returned properly. -
Authorization: (Often required) This provides credentials (API keys or tokens) to prove your identity and permission to access the API. This is extremely important and this is what protects API’s from attackers.
Failing to include the necessary headers can lead to your request being rejected.
It’s like showing up at the restaurant without your wallet; they won’t serve you!
Using the right headers ensures seamless communication between your application and the API reducing errors and ensuring you get what you need.
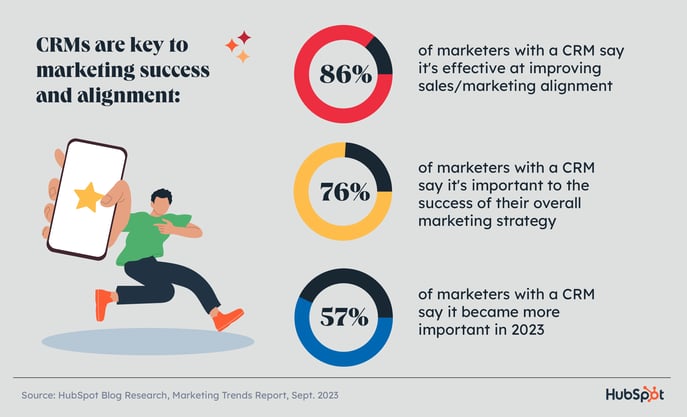
This is super important and will prevent a lot of time-wasting debugging later.
Step 4: Authentication: API Keys and Access Tokens
Most APIs require authentication to prevent unauthorized access.
This usually involves using an API key or an access token which is like a password that grants you access to the API’s resources.
The API documentation will tell you how to obtain and use these credentials.
Without them you’ll likely get a “401 Unauthorized” error.
This is a critical step; secure your API’s!
Many API’s use different types of authentication methods like API Keys OAuth 2.0 JWT’s Basic Authentication and many more.
Each of them have their own advantages and disadvantages and using the right one for your API is important.

Consider your security requirements when choosing one!
Step 5: Receive and Process the Response
After sending your request the API sends back a response.
This typically includes a status code (e.g.
200 OK 404 Not Found 500 Internal Server Error) and the requested data.
A 200 code means everything went smoothly; other codes indicate errors that you’ll need to investigate and fix.
The data usually comes in JSON or XML format which you’ll need to parse (interpret) to use it in your application.

Error handling is a key part of working with APIs.

Be prepared to deal with various error codes and handle them gracefully.
You don’t want your application to crash just because the API had a hiccup! Its very important to use robust error handling techniques to make sure your applications handle unexpected events.

Testing Your API Calls: Make Sure It Works!
Before integrating an API into your application thorough testing is essential.

You wouldn’t build a house without checking the foundation right?
There are several tools available for testing API calls including Postman (a very popular choice) curl (a command-line tool) and online API testing platforms.
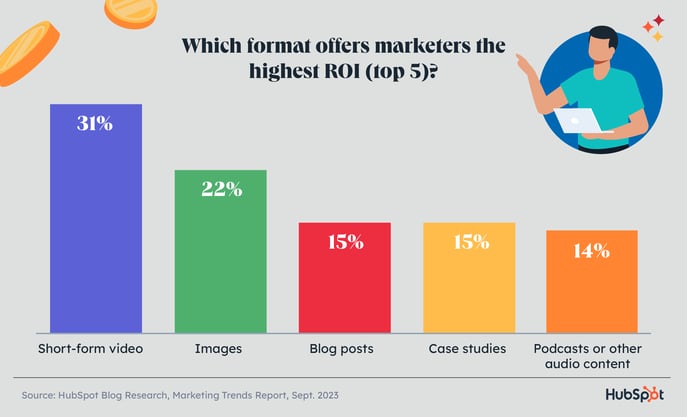
These tools allow you to send requests and inspect the responses ensuring the API behaves as expected.
Pay close attention to the response codes.
If you get anything other than a 2xx (success) code you’ve got a problem to solve.
Testing also involves checking that the API’s documentation is accurate.
Many API’s have thorough documentation and testing to make sure everything is working correctly.
This step is crucial to ensure that the API does what you think it does!
API Call Best Practices: Pro Tips for Success
Here are a few tips to make your API interactions smoother and more efficient:
Check our top articles on API Calls: What They Are & How to Make Them in 5 Easy Steps
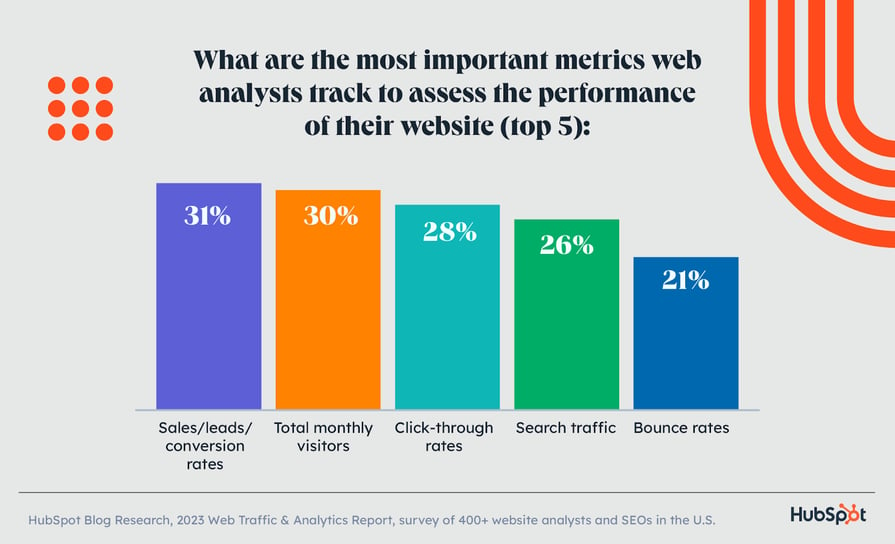
-
Read the documentation carefully: APIs come with instructions. Follow them!
-
Rate limiting: Many APIs limit the number of requests you can make within a given time. Respect these limits to avoid getting your requests blocked.
Ready to level up your dev game? 🚀 This guide breaks down API calls like a pro. Want the cheat sheet to master them? Check out this awesome resource! 💯
-
Error handling: Build error handling into your application to gracefully manage unexpected situations.
-
Security: Protect your API keys and tokens. Don’t hardcode them directly into your application—use environment variables or a secure configuration system.
-
Caching: Cache frequently accessed data to reduce the load on the API and improve performance. This might involve using a caching library or building custom caching mechanisms.
API calls are a fundamental part of modern software development.
They’re powerful but don’t let the technical jargon intimidate you.

Ready to level up your dev game? 🚀 This guide breaks down API calls like a pro. Want the cheat sheet to master them? Check out this awesome resource! 💯

With some practice and a methodical approach you can master them and build some amazing things! Good luck and happy coding!
